C Functions
C structures, c reference, c structures (structs).
Structures (also called structs) are a way to group several related variables into one place. Each variable in the structure is known as a member of the structure.
Unlike an array , a structure can contain many different data types (int, float, char, etc.).

Create a Structure
You can create a structure by using the struct keyword and declare each of its members inside curly braces:
To access the structure, you must create a variable of it.
Use the struct keyword inside the main() method, followed by the name of the structure and then the name of the structure variable:
Create a struct variable with the name "s1":
Access Structure Members
To access members of a structure, use the dot syntax ( . ):
Now you can easily create multiple structure variables with different values, using just one structure:
Advertisement
What About Strings in Structures?
Remember that strings in C are actually an array of characters, and unfortunately, you can't assign a value to an array like this:
An error will occur:
However, there is a solution for this! You can use the strcpy() function and assign the value to s1.myString , like this:
Simpler Syntax
You can also assign values to members of a structure variable at declaration time, in a single line.
Just insert the values in a comma-separated list inside curly braces {} . Note that you don't have to use the strcpy() function for string values with this technique:
Note: The order of the inserted values must match the order of the variable types declared in the structure (13 for int, 'B' for char, etc).
Copy Structures
You can also assign one structure to another.
In the following example, the values of s1 are copied to s2:
Modify Values
If you want to change/modify a value, you can use the dot syntax ( . ).
And to modify a string value, the strcpy() function is useful again:
Modifying values are especially useful when you copy structure values:
Ok, so, how are structures useful?
Imagine you have to write a program to store different information about Cars, such as brand, model, and year. What's great about structures is that you can create a single "Car template" and use it for every cars you make. See below for a real life example.
Real-Life Example
Use a structure to store different information about Cars:
C Exercises
Test yourself with exercises.
Fill in the missing part to create a Car structure:
Start the Exercise

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
Next: Unions , Previous: Overlaying Structures , Up: Structures [ Contents ][ Index ]
15.13 Structure Assignment
Assignment operating on a structure type copies the structure. The left and right operands must have the same type. Here is an example:
Notionally, assignment on a structure type works by copying each of the fields. Thus, if any of the fields has the const qualifier, that structure type does not allow assignment:
See Assignment Expressions .
When a structure type has a field which is an array, as here,
structure assigment such as r1 = r2 copies array fields’ contents just as it copies all the other fields.
This is the only way in C that you can operate on the whole contents of a array with one operation: when the array is contained in a struct . You can’t copy the contents of the data field as an array, because
would convert the array objects (as always) to pointers to the zeroth elements of the arrays (of type struct record * ), and the assignment would be invalid because the left operand is not an lvalue.
cppreference.com
Struct declaration.
(C11) | ||||
Miscellaneous | ||||
(C11) | ||||
(C23) |
A struct is a type consisting of a sequence of members whose storage is allocated in an ordered sequence (as opposed to union, which is a type consisting of a sequence of members whose storage overlaps).
The type specifier for a struct is identical to the union type specifier except for the keyword used:
Syntax Explanation Forward declaration Keywords Notes Example Defect reports References See also |
[ edit ] Syntax
attr-spec-seq (optional) name (optional) struct-declaration-list | (1) | ||||||||
attr-spec-seq (optional) name | (2) | ||||||||
name | - | the name of the struct that's being defined |
struct-declaration-list | - | any number of variable declarations, declarations, and declarations. Members of incomplete type and members of function type are not allowed (except for the flexible array member described below) |
attr-spec-seq | - | (C23)optional list of , applied to the struct type |
[ edit ] Explanation
Within a struct object, addresses of its elements (and the addresses of the bit-field allocation units) increase in order in which the members were defined. A pointer to a struct can be cast to a pointer to its first member (or, if the member is a bit-field, to its allocation unit). Likewise, a pointer to the first member of a struct can be cast to a pointer to the enclosing struct. There may be unnamed padding between any two members of a struct or after the last member, but not before the first member. The size of a struct is at least as large as the sum of the sizes of its members.
If a struct defines at least one named member, it is allowed to additionally declare its last member with incomplete array type. When an element of the flexible array member is accessed (in an expression that uses operator or with the flexible array member's name as the right-hand-side operand), then the struct behaves as if the array member had the longest size fitting in the memory allocated for this object. If no additional storage was allocated, it behaves as if an array with 1 element, except that the behavior is undefined if that element is accessed or a pointer one past that element is produced. Initialization and the assignment operator ignore the flexible array member. sizeof omits it, but may have more trailing padding than the omission would imply. Structures with flexible array members (or unions who have a recursive-possibly structure member with flexible array member) cannot appear as array elements or as members of other structures. s { int n; double d[]; }; // s.d is a flexible array member struct s t1 = { 0 }; // OK, d is as if double d[1], but UB to access struct s t2 = { 1, { 4.2 } }; // error: initialization ignores flexible array // if sizeof (double) == 8 struct s *s1 = (sizeof (struct s) + 64); // as if d was double d[8] struct s *s2 = (sizeof (struct s) + 40); // as if d was double d[5] s1 = (sizeof (struct s) + 10); // now as if d was double d[1]. Two bytes excess. double *dp = &(s1->d[0]); // OK *dp = 42; // OK s1->d[1]++; // Undefined behavior. 2 excess bytes can't be accessed // as double. s2 = (sizeof (struct s) + 6); // same, but UB to access because 2 bytes are // missing to complete 1 double dp = &(s2->d[0]); // OK, can take address just fine *dp = 42; // undefined behavior *s1 = *s2; // only copies s.n, not any element of s.d // except those caught in sizeof (struct s) | (since C99) |
Similar to union, an unnamed member of a struct whose type is a struct without name is known as . Every member of an anonymous struct is considered to be a member of the enclosing struct or union, keeping their structure layout. This applies recursively if the enclosing struct or union is also anonymous. v { union // anonymous union { struct { int i, j; }; // anonymous structure struct { long k, l; } w; }; int m; } v1; v1.i = 2; // valid v1.k = 3; // invalid: inner structure is not anonymous v1.w.k = 5; // validSimilar to union, the behavior of the program is undefined if struct is defined without any named members (including those obtained via anonymous nested structs or unions). | (since C11) |
[ edit ] Forward declaration
A declaration of the following form
attr-spec-seq (optional) name | |||||||||
hides any previously declared meaning for the name name in the tag name space and declares name as a new struct name in current scope, which will be defined later. Until the definition appears, this struct name has incomplete type .
This allows structs that refer to each other:
Note that a new struct name may also be introduced just by using a struct tag within another declaration, but if a previously declared struct with the same name exists in the tag name space , the tag would refer to that name
[ edit ] Keywords
[ edit ] notes.
See struct initialization for the rules regarding the initializers for structs.
Because members of incomplete type are not allowed, and a struct type is not complete until the end of the definition, a struct cannot have a member of its own type. A pointer to its own type is allowed, and is commonly used to implement nodes in linked lists or trees.
Because a struct declaration does not establish scope , nested types, enumerations and enumerators introduced by declarations within struct-declaration-list are visible in the surrounding scope where the struct is defined.
[ edit ] Example
Possible output:
[ edit ] Defect reports
The following behavior-changing defect reports were applied retroactively to previously published C standards.
DR | Applied to | Behavior as published | Correct behavior |
---|---|---|---|
C11 | members of anonymous structs/unions were considered members of the enclosing struct/union | they retain their memory layout |
[ edit ] References
- C23 standard (ISO/IEC 9899:2024):
- 6.7.2.1 Structure and union specifiers (p: TBD)
- C17 standard (ISO/IEC 9899:2018):
- 6.7.2.1 Structure and union specifiers (p: 81-84)
- C11 standard (ISO/IEC 9899:2011):
- 6.7.2.1 Structure and union specifiers (p: 112-117)
- C99 standard (ISO/IEC 9899:1999):
- 6.7.2.1 Structure and union specifiers (p: 101-104)
- C89/C90 standard (ISO/IEC 9899:1990):
- 3.5.2.1 Structure and union specifiers
[ edit ] See also
- struct and union member access
- struct initialization
for Class declaration |
- Recent changes
- Offline version
- What links here
- Related changes
- Upload file
- Special pages
- Printable version
- Permanent link
- Page information
- In other languages
- This page was last modified on 6 January 2024, at 23:45.
- Privacy policy
- About cppreference.com
- Disclaimers

Home » Learn C Programming from Scratch » C Structure
C Structure
Summary : in this tutorial, you will learn how to define a new type called C structure that allows you to wrap related variables with different types into a single entity.
Introduction to C structure
When you design software, it is important to choose an optimal way to represent the data that the program processes.
In simple cases, scalar variables and arrays are sufficient. However, in practical applications, you need a new form of variable that reflects the real-world data.
For example, you may want to reference an address that holds multiple fields including house number, street, zip code, state, and country.
To do it, C provides you with the structure type. A structure allows you to wrap related variables with different data types into a single variable.
Defining structure
To define a structure, you use the struct keyword. The following shows the syntax for defining a structure:
In this syntax:
- First, use the struct keyword followed by the structure name.
- Second, specify the elements of the structure including type and name. The elements of the structure are also called fields.
For example, the following defines the address structure:
The address structure includes house number, street name, city, state, zip code, and country.
Declaring structure variables
The above example defines the address structure without declaring any structure variables. C provides you with two ways to declare structure variables:
1) Declaring structure variables with the structure definition
The follownig shows the syntax for declaring structure variables with the structure definition:
In this syntax, you can omit the structure name like this:
For example, you can define the address structure and declare two structure variables:
In this example, the home_address and business_address are the structure variables. Alternatively, you can declare the structure variables as follows:
2) Declaring structure variables after defining the structure
The following shows the syntax for defining a structure and its variables:
In this syntax, you separate the structure definition from the variable declaration. For example:
Initializing structure variables
Like a regular variable, you can initialize a structure variable. The following example shows the syntax for initializing a structure variable:
For example, you can define a structure, declare a structure variable, and initialize the structure variable like this:
Accessing structure members
To access a structure member, you use the dot operator ( . ) as follows:
The following programm print out the fields of the address structure:
Structures that contain other structures
As mentioned earlier, a structure may contain another structure. For example, you can define the customer structure that contains the address structures:
In this example, the customer structure contains two members, shipping_address and billing_address which are also the structures.
When a structure contains a structure, you use the dot operator ( . ) to access the fields of the nested structure.
The following example defines the customer structure and variable ( john ). It then copies the string "San Fransisco" to the city of the shipping address of the customer:
Assigning a structure into another structure
C allows you to assign a structure to another by using the assignment operator ( = ):
Notice that some old C compilers may not support structure assignment. If this is the case, you have to assign each structure member individually.
The following program illustrates how to assign the business_address structure to the home_address structure using the assignment operator ( = ):
C structure type and the sizeof operator
To get the size of a structure, you cannot just simply add up the size of its fields. Instead, you use the sizeof() operator like the following example:
The sizeof() operator returns the size of a structure that is always bigger than the size of all the structure members.
The reason is that the compilers always need to pad the structure members so that each member can be accessed faster.
Structure shorthand with typedef keyword
The typedef keyword allows you to assign a new type name to an existing type. For example, you can assign a structure a new type name and use that type to declare a variable.
The following example uses the typedef keyword to define the address structure type:
The address is a new type now. So you can use it to declare variables like this:
The typedef makes your code less verbose and more concise.
C structure example
The following program illustrates how to use the structure to read the product information including name and quantity from the command line and display the total quantity of all the products:
To change the number of products that you want to enter, you need to change the SIZE constant.
- A structure type combines multiple related fields with different types.
- Use the struct keyword to define a structure.
- Use the dot operator (.) to access the members of the structure.
- Course List
- Introduction to C
- Environment Setup
- First C Application
- Basic Syntax
- Variables & Constants
- if, else, switch, ternary
- while, do while & for loops
- Jump statement goto
- Intermediate
- Dynamic Memory Management
- Preprocessor directives & macros
C Structs (structures) Tutorial
In this C tutorial we learn how to define our own types in C with structs.
We cover how to define a struct, declare a struct variable, assign data to a struct member and how to assign data to a struct pointer member.
- What is a struct
How to define a struct
How to declare a struct variable, how to access and assign data to a struct property member, how to access and assign data to a struct pointer.
A struct is a user-defined type that can store one or more variables of different types. We use structs to model our application after entities in the real world, grouping related code.
As an example, let’s consider a player character in an first person shooter game. Each player has a list of properties associated with them.
- Player name
- Primary weapon
- Secondary weapon
Our first thought would be to throw all these properties into an array, however, the properties have different types.
We could create individual variables, but what if the player wants to play a co-op match where more than one player’s data needs to be stored.
This is where a struct is useful. A struct allows us to group all of these properties together as a single unit, and then create multiple units.
For example, we group the player properties into a struct called Player. We can then create multiple players of type Player, each with different values.
To define a struct, we use the keyword struct , followed by a name and a code block. Inside the code block we define the struct properties.
Note that a struct’s code block is terminated with a semicolon.
In the example above, we define the struct above the main() function. If we define it below the main() function the compiler will raise an error.
The C languages is organized around one pass compilation, which means it scans the document only once from top to bottom before compiling.
If we define a struct below a point where it’s used, the main() function for example, the compiler will see an undefined type because it doesn’t know about the struct yet.
In functions we get around this problem with a prototype at the top of the document. But, structs don’t have prototypes so we define them above the point they would be used.
Typically, a struct would go into an external file, something like player.h and be included at the top of the document like we do with the stdio.h file.
When we define a structure, it becomes a type, like int or char. Therefore, we need a variable that is of the struct’s type.
There are two ways to create a struct variable:
- After the structure definition.
- With the structure definition.
Let’s take a look at declaring a struct variable the struct’s definition first.
We write the struct keyword, followed by the struct name as the type. Lastly, we give our variable a name.
In the example above, we create a new struct variable with the name p1 of type Player . We will be able to access the struct’s properties with the p1 variable.
We can also declare a variable for the struct when we define the struct itself.
To do this we add the variable name after the closing curly brace of the struct, but before the semicolon terminator.
In the example above, we declare three variables after the struct’s closing curly brace. When declaring multiple struct variables, we can just separate them with a comma.
If we’ve declared a struct variable, we can use it to access any property inside the struct with dot notation.
Dot notation is separating the variable name and property name with a dot operator ( . ).
To assign data we use the assignment operator followed by the data we want to store in the property.
In the example above, we add ammuntion to our ammo property and then print it to the console.
When we assign data to a property, it doesn’t affect the struct itself, only the p1 instance of it .
Since we can create pointers to any type, and a structure is a type, we can also create pointers to structs.
However, we are not allowed to access the structure pointer member by using the dot operator. Instead, we use arrow notation ( -> ) to access the structure member.
Before we are able to access the property, we will need to initialize the pointer to the struct through dynamic memory allocation .
In the example above, we create a struct pointer p1 . Then we initialize the pointer as a struct pointer with the appropriate size. Finally, we access the members of p1 with arrow notation ( -> ).
Remember to release the memory afterwards with the free() function .
- Summary: Points to remember
- A struct is a user-defined type that can hold multiple different types of variables.
- A struct variable can be declared either on its own or as part of the struct definition.
- We access a struct property member with dot notation ( . ).
- We access a struct pointer member with arrow notation ( -> ).
- Define a struct
- Define a struct variable
- Access struct members
- Access struct pointer member
Structured data types in C - Struct and Typedef Explained with Examples
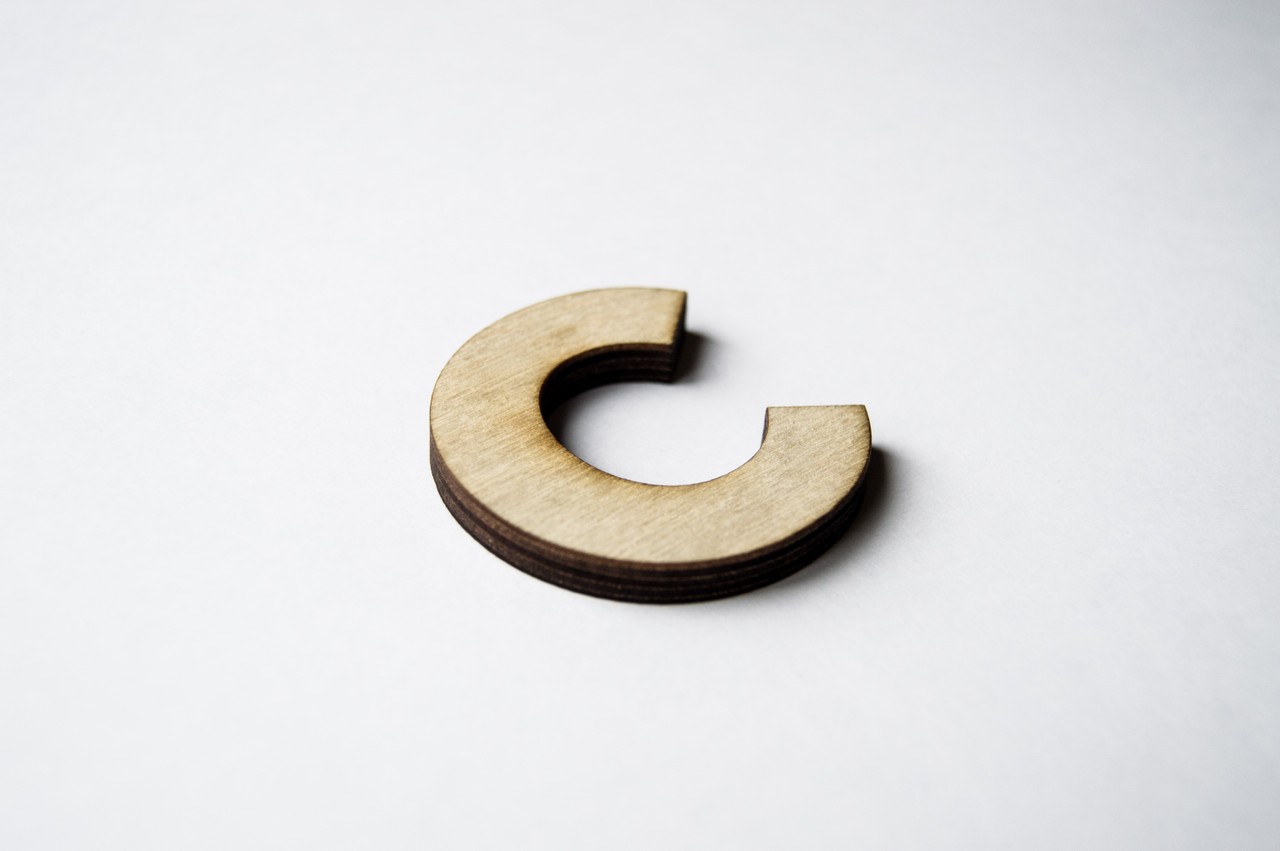
During your programming experience you may feel the need to define your own type of data. In C this is done using two keywords: struct and typedef . Structures and unions will give you the chance to store non-homogenous data types into a single collection.
Declaring a new data type
After this little code student will be a new reserved keyword and you will be able to create variables of type student . Please mind that this new kind of variable is going to be structured which means that defines a physically grouped list of variables to be placed under one name in a block of memory.
New data type usage
Let’s now create a new student variable and initialize its attributes:
As you can see in this example you are required to assign a value to all variables contained in your new data type. To access a structure variable you can use the point like in stu.name . There is also a shorter way to assign values to a structure:
Or if you prefer to set it’s values following a different order:
Unions vs Structures
Unions are declared in the same was as structs, but are different because only one item within the union can be used at any time.
You should use union in such case where only one condition will be applied and only one variable will be used. Please do not forget that we can use our brand new data type too:

A few more tricks
- When you create a pointer to a structure using the & operator you can use the special -> infix operator to deference it. This is very used for example when working with linked lists in C
- The new defined type can be used just as other basic types for almost everything. Try for example to create an array of type student and see how it works.
- Structs can be copied or assigned but you can not compare them!
More Information:
- The C Beginner's Handbook: Learn C Programming Language basics in just a few hours
- Data Types in C - Integer, Floating Point, and Void Explained
- malloc in C: Dynamic Memory Allocation in C Explained
If you read this far, thank the author to show them you care. Say Thanks
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
Intro to C for CS31 Students
Part 2: structs & pointers.
- Pointers and Functions C style "pass by referece"
- Dynamic Memory Allocation (malloc and free)
- Pointers to Structs
Defining a struct type
Declaring variables of struct types, accessing field values, passing structs to functions, rules for using pointer variables.
- Next, initialize the pointer variable (make it point to something). Pointer variables store addresses . Initialize a pointer to the address of a storage location of the type to which it points. One way to do this is to use the ampersand operator on regular variable to get its address value: int x; char ch; ptr = &x; // ptr get the address of x, pointer "points to" x ------------ ------ ptr | addr of x|--------->| ?? | x ------------ ------ cptr = &ch; // ptr get the address of ch, pointer "points to" ch cptr = &x; // ERROR! cptr can hold a char address only (it's NOT a pointer to an int) All pointer variable can be set to a special value NULL . NULL is not a valid address but it is useful for testing a pointer variable to see if it points to a valid memory address before we access what it points to: ptr = NULL; ------ ------ cptr = NULL; ptr | NULL |-----| cptr | NULL |----| ------ ------
- Use *var_name to dereference the pointer to access the value in the location that it points to. Some examples: int *ptr1, *ptr2, x, y; x = 8; ptr1 = NULL; ptr2 = &x; ------------ ------ ptr2 | addr of x|--------->| 8 | x ------------ ------ *ptr2 = 10; // dereference ptr2: "what ptr2 points to gets 10" ------------ ----- ptr2 | addr of x|--------->| 10 | x ------------ ----- y = *ptr2 + 3; // dereference ptr2: "y gets what ptr2 points to plus 3" ----- ----- ptr1 = ptr2; // ptr1 gets address value stored in ptr2 ------------ ----- ptr2 | addr of x |--------->| 10 | x ------------ ----- /\ ------------ | ptr1 | addr of x |-------------- ------------ // TODO: finish tracing through this code and show what is printed *ptr1 = 100; ptr1 = &y; *ptr1 = 80; printf("x = %d y = %d\n", x, y); printf("x = %d y = %d\n", *ptr2, *ptr1);
- and what does this mean with respect to the value of each argument after the call?
- Implement a program with a swap function that swaps the values stored in its two arguments. Make some calls to it in main to test it out.
malloc and free
Pointers, the heap, and functions, linked lists in c.

- C Programming Tutorial
- Basics of C
- C - Overview
- C - Features
- C - History
- C - Environment Setup
- C - Program Structure
- C - Hello World
- C - Compilation Process
- C - Comments
- C - Keywords
- C - Identifiers
- C - User Input
- C - Basic Syntax
- C - Data Types
- C - Variables
- C - Integer Promotions
- C - Type Conversion
- C - Type Casting
- C - Booleans
- Constants and Literals in C
- C - Constants
- C - Literals
- C - Escape sequences
- C - Format Specifiers
- Operators in C
- C - Operators
- C - Arithmetic Operators
- C - Relational Operators
- C - Logical Operators
- C - Bitwise Operators
- C - Assignment Operators
- C - Unary Operators
- C - Increment and Decrement Operators
- C - Ternary Operator
- C - sizeof Operator
- C - Operator Precedence
- C - Misc Operators
- Decision Making in C
- C - Decision Making
- C - if statement
- C - if...else statement
- C - nested if statements
- C - switch statement
- C - nested switch statements
- C - While loop
- C - For loop
- C - Do...while loop
- C - Nested loop
- C - Infinite loop
- C - Break Statement
- C - Continue Statement
- C - goto Statement
- Functions in C
- C - Functions
- C - Main Function
- C - Function call by Value
- C - Function call by reference
- C - Nested Functions
- C - Variadic Functions
- C - User-Defined Functions
- C - Callback Function
- C - Return Statement
- C - Recursion
- Scope Rules in C
- C - Scope Rules
- C - Static Variables
- C - Global Variables
- Arrays in C
- C - Properties of Array
- C - Multi-Dimensional Arrays
- C - Passing Arrays to Function
- C - Return Array from Function
- C - Variable Length Arrays
- Pointers in C
- C - Pointers
- C - Pointers and Arrays
- C - Applications of Pointers
- C - Pointer Arithmetics
- C - Array of Pointers
- C - Pointer to Pointer
- C - Passing Pointers to Functions
- C - Return Pointer from Functions
- C - Function Pointers
- C - Pointer to an Array
- C - Pointers to Structures
- C - Chain of Pointers
- C - Pointer vs Array
- C - Character Pointers and Functions
- C - NULL Pointer
- C - void Pointer
- C - Dangling Pointers
- C - Dereference Pointer
- C - Near, Far and Huge Pointers
- C - Initialization of Pointer Arrays
- C - Pointers vs. Multi-dimensional Arrays
- Strings in C
- C - Strings
- C - Array of Strings
- C - Special Characters
- C Structures and Unions
- C - Structures
- C - Structures and Functions
- C - Arrays of Structures
- C - Self-Referential Structures
- C - Lookup Tables
- C - Dot (.) Operator
- C - Enumeration (or enum)
- C - Structure Padding and Packing
- C - Nested Structures
- C - Anonymous Structure and Union
- C - Bit Fields
- C - Typedef
- File Handling in C
- C - Input & Output
- C - File I/O (File Handling)
- C Preprocessors
- C - Preprocessors
- C - Pragmas
- C - Preprocessor Operators
- C - Header Files
- Memory Management in C
- C - Memory Management
- C - Memory Address
- C - Storage Classes
- Miscellaneous Topics
- C - Error Handling
- C - Variable Arguments
- C - Command Execution
- C - Math Functions
- C - Static Keyword
- C - Random Number Generation
- C - Command Line Arguments
- C Programming Resources
- C - Questions & Answers
- C - Quick Guide
- C - Cheat Sheet
- C - Useful Resources
- C - Discussion
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
Structures in C
A structure in C is a derived or user-defined data type. We use the keyword struct to define a custom data type that groups together the elements of different types. The difference between an array and a structure is that an array is a homogenous collection of similar types, whereas a structure can have elements of different types stored adjacently and identified by a name.
We are often required to work with values of different data types having certain relationships among them. For example, a book is described by its title (string), author (string), price (double), number of pages (integer), etc. Instead of using four different variables, these values can be stored in a single struct variable.
Declare (Create) a Structure
You can create (declare) a structure by using the "struct" keyword followed by the structure_tag (structure name) and declare all of the members of the structure inside the curly braces along with their data types.
To define a structure, you must use the struct statement. The struct statement defines a new data type, with more than one member.
Syntax of Structure Declaration
The format (syntax) to declare a structure is as follows −
The structure tag is optional and each member definition is a normal variable definition, such as "int i;" or "float f;" or any other valid variable definition.
At the end of the structure's definition, before the final semicolon, you can specify one or more structure variables but it is optional .
In the following example we are declaring a structure for Book to store the details of a Book −
Here, we declared the structure variable book1 at the end of the structure definition. However, you can do it separately in a different statement.
Structure Variable Declaration
To access and manipulate the members of the structure, you need to declare its variable first. To declare a structure variable, write the structure name along with the "struct" keyword followed by the name of the structure variable. This structure variable will be used to access and manipulate the structure members.
The following statement demonstrates how to declare (create) a structure variable
Usually, a structure is declared before the first function is defined in the program, after the include statements. That way, the derived type can be used for declaring its variable inside any function.
Structure Initialization
The initialization of a struct variable is done by placing the value of each element inside curly brackets.
The following statement demonstrates the initialization of structure
Accessing the Structure Members
To access the members of a structure, first, you need to declare a structure variable and then use the dot (.) operator along with the structure variable.
The four elements of the struct variable book1 are accessed with the dot (.) operator . Hence, "book1.title" refers to the title element, "book1.author" is the author name, "book1.price" is the price, "book1.pages" is the fourth element (number of pages).
Take a look at the following example −
Run the code and check its output −
In the above program, we will make a small modification. Here, we will put the type definition and the variable declaration together, like this −
Note that if you a declare a struct variable in this way, then you cannot initialize it with curly brackets. Instead, the elements need to be assigned individually.
When you execute this code, it will produce the following output −
Copying Structures
The assignment (=) operator can be used to copy a structure directly. You can also use the assignment operator (=) to assign the value of the member of one structure to another.
Let's have two struct book variables, book1 and book2 . The variable book1 is initialized with declaration, and we wish to assign the same values of its elements to that of book2 .
We can assign individual elements as follows −
Note the use of strcpy() function to assign the value to a string variable instead of using the "= operator".
You can also assign book1 to book2 so that all the elements of book1 are respectively assigned to the elements of book2. Take a look at the following program code −
Structures as Function Arguments
You can pass a structure as a function argument in the same way as you pass any other variable or pointer.
Take a look at the following program code. It demonstrates how you can pass a structure as a function argument −
When the above code is compiled and executed, it produces the following result −
Pointers to Structures
You can define pointers to structures in the same way as you define pointers to any other variable.
Declaration of Pointer to a Structure
You can declare a pointer to a structure (or structure pointer) as follows −
Initialization of Pointer to a Structure
You can store the address of a structure variable in the above pointer variable struct_pointer . To find the address of a structure variable, place the '&' operator before the structure's name as follows −
Let's store the address of a struct variable in a struct pointer variable.
Accessing Members Using Pointer to a Structure
To access the members of a structure using a pointer to that structure, you must use the → operator as follows −
C defines the → symbol to be used with struct pointer as the indirection operator (also called struct dereference operator ). It helps to access the elements of the struct variable to which the pointer reference to.
In this example, strptr is a pointer to struct book book1 variable. Hence, strrptr→title returns the title, just like book1.title does.
When you run this code, it will produce the following output −
Note: The dot (.) operator is used to access the struct elements via the struct variable. To access the elements via its pointer, we must use the indirection (->) operator
A struct variable is like a normal variable of primary type, in the sense that you can have an array of struct, you can pass the struct variable to a function, as well as return a struct from a function.
You may have noted that you need to prefix "struct type" to the name of the variable or pointer at the time of declaration. This can be avoided by creating a shorthand notation with the help of typedef keyword, which we will explain in a subsequent chapter.
Structures are used in different applications such as databases, file management applications, and for handling complex data structures such as tree and linked lists.
Bit Fields allow the packing of data in a structure. This is especially useful when memory or data storage is at a premium. Typical examples include −
- Packing several objects into a machine word, for example, 1-bit flags can be compacted.
- Reading external file formats − non-standard file formats could be read in, for example, 9-bit integers.
Declaration
C allows us to do this in a structure definition by putting :bit length after the variable. For example −
Here, the packed_struct contains 6 members: Four 1 bit flags f1..f3, a 4-bit type and a 9-bit my_int.
C automatically packs the above bit fields as compactly as possible, provided that the maximum length of the field is less than or equal to the integer word length of the computer. If this is not the case, then some compilers may allow memory overlap for the fields while others would store the next field in the next word.
Learn C practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn c interactively, c introduction.
- Getting Started with C
- Your First C Program
C Fundamentals
- C Variables, Constants and Literals
- C Data Types
- C Input Output (I/O)
- C Programming Operators
C Flow Control
- C if...else Statement
- C while and do...while Loop
- C break and continue
- C switch Statement
- C goto Statement
- C Functions
- C User-defined functions
- Types of User-defined Functions in C Programming
- C Recursion
- C Storage Class
C Programming Arrays
- C Multidimensional Arrays
Pass arrays to a function in C
C Programming Pointers
- Relationship Between Arrays and Pointers
- C Pass Addresses and Pointers
- C Dynamic Memory Allocation
- C Array and Pointer Examples
- C Programming Strings
- String Manipulations In C Programming Using Library Functions
- String Examples in C Programming
C Structure and Union
C structs and Pointers
C Structure and Function
C programming files.
- C File Handling
C Files Examples
C Additional Topics
- C Keywords and Identifiers
- C Precedence And Associativity Of Operators
- C Bitwise Operators
- C Preprocessor and Macros
- C Standard Library Functions
C Tutorials
- Add Two Complex Numbers by Passing Structure to a Function
- Store Information of Students Using Structure
- Store Information of a Student Using Structure
- Add Two Distances (in inch-feet system) using Structures
Similar to variables of built-in types, you can also pass structure variables to a function.
Passing structs to functions
We recommended you to learn these tutorials before you learn how to pass structs to functions.
- C structures
- C functions
- User-defined Function
Here's how you can pass structures to a function
Here, a struct variable s1 of type struct student is created. The variable is passed to the display() function using display(s1); statement.
Return struct from a function
Here's how you can return structure from a function:
Here, the getInformation() function is called using s = getInformation(); statement. The function returns a structure of type struct student . The returned structure is displayed from the main() function.
Notice that, the return type of getInformation() is also struct student .
Passing struct by reference
You can also pass structs by reference (in a similar way like you pass variables of built-in type by reference). We suggest you to read pass by reference tutorial before you proceed.
During pass by reference, the memory addresses of struct variables are passed to the function.
In the above program, three structure variables c1 , c2 and the address of result is passed to the addNumbers() function. Here, result is passed by reference.
When the result variable inside the addNumbers() is altered, the result variable inside the main() function is also altered accordingly.
Table of Contents
- Passing structure to a function
- Return struct from a function
- Passing struct by reference
Sorry about that.
Our premium learning platform, created with over a decade of experience and thousands of feedbacks .
Learn and improve your coding skills like never before.
- Interactive Courses
- Certificates
- 2000+ Challenges
Related Tutorials
- C Data Types
- C Operators
- C Input and Output
- C Control Flow
- C Functions
- C Preprocessors
- C File Handling
- C Cheatsheet
- C Interview Questions
Operations on struct variables in C
In C, the only operation that can be applied to struct variables is assignment. Any other operation (e.g. equality check) is not allowed on struct variables. For example, program 1 works without any error and program 2 fails in compilation.
Please Login to comment...
Similar reads.
- C-Struct-Union-Enum
- OpenAI o1 AI Model Launched: Explore o1-Preview, o1-Mini, Pricing & Comparison
- How to Merge Cells in Google Sheets: Step by Step Guide
- How to Lock Cells in Google Sheets : Step by Step Guide
- PS5 Pro Launched: Controller, Price, Specs & Features, How to Pre-Order, and More
- #geekstreak2024 – 21 Days POTD Challenge Powered By Deutsche Bank
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
how to assign multiple values into a struct at once?
I can do this on initialization for a struct Foo:
but, I can't do this:
So, two questions:
- Why can't I do the latter, is the former a special constructor for initialization only?
How can I do something similar to the second example, i.e. declare a bunch of variables for a struct in a single line of code after it's already been initialized? I'm trying to avoid having to do this for large structs with many variables:

- 4 " is the former a special constructor for initialization only? " Yes, precisely -- it's called aggregate initialization . – ildjarn Commented Feb 13, 2012 at 23:52
- 14 C or C++? Pick one. – Lightness Races in Orbit Commented Feb 13, 2012 at 23:53
- 2 C(++) remains very primitive in some areas. – Alexey Frunze Commented Feb 13, 2012 at 23:54
- 3 @jim : They're different languages and consequently warrant different questions. For this question, choose a single language please. – ildjarn Commented Feb 14, 2012 at 0:05
- 4 @jim Asking for solutions in C and C++ makes it two different questions, albeit with common themes. You are only meant to ask one question at a time. I gave an answer for C, but when I realised that the question had both tags I deleted it. – David Heffernan Commented Feb 14, 2012 at 0:24
6 Answers 6
This will work if you have a good compiler (e.g. GCC).
Update: In modern versions of C (but not C++), you can also use a compound literal with designated initializers, which looks like this:
The name right after the "." should be the name of the structure member you wish to initialize. These initializers can appear in any order, and any member that is not specified explicitly will get initialized to zero.
- very cool, unfortunately, doesn't work on my (bad) compiler: MSVC2008. :( – jim Commented Feb 13, 2012 at 23:57
- @jim : VC++ 2008 isn't a "bad" compiler (though it certainly isn't the best); it just doesn't support C++11, which is needed for what you want. – ildjarn Commented Feb 13, 2012 at 23:59
- @ildjarn: i know, that's why i put "bad" in parethesis (should have been in quotes i guess). i was poking at David's implication, that if you can't compile the above, you have a "bad" compiler. i'm not savvy enough to have an opinion either way. – jim Commented Feb 14, 2012 at 0:06
- 4 @jim - the aggregate assignment syntax shown by David was introduced with the 1999 standard (and yes, with gcc you have to specify --std=c99 or later for it to be recognized). Unfortunately, Microsoft has decided not to support C99 in the VS suite. It'll be interesting to see if they decide to support C11. – John Bode Commented Feb 14, 2012 at 0:15
- 2 Combined with @JohnHumpreys answer below, you could do something like: foo = (Foo){.bunch = bunch, .of = of, .things = things, .initialized = initialized}; – kevinarpe Commented Apr 4, 2022 at 15:17
The first is an aggregate initializer - you can read up on those and tagged initializers at this solution:
What is tagged structure initialization syntax?
It is a special initialization syntax, and you can't do something similar after initialization of your struct. What you can do is provide a member (or non-member) function to take your series of values as parameters which you then assign within the member function - that would allow you to accomplish this after the structure is initialized in a way that is equally concise (after you've written the function the first time of course!)
- I found this thread asking the same question: stackoverflow.com/questions/1705147/… . However, all of the answers, along with Davids (which I really liked and upvoted), seemed to be compiler specific. This is something I can actually implement on my platform, so I chose this as the answer. Thanks all. – jim Commented Feb 14, 2012 at 1:42
In C++11 you can perform multiple assignment with "tie" (declared in the tuple header)
If your right hand expression is of fixed size and you only need to get some of the elements, you can use the ignore placeholder with tie
If you find the syntax std::tie(f.a, f.b, f.c) too code cluttering you could have a member function returning that tuple of references
All this ofcourse assuming that overloading the assignment operator is not an option because your struct is not constructible by such sequence of values, in which case you could say
- 1 what about performances? Is there an overhead doing such a thing? or is it resolved at compile time? – Raffaello Commented Mar 21, 2020 at 15:42
Memory Footprint - Here is an interesting i386 addition.
After much hassle, using optimization and memcpy seems to generate the smallest footprint using i386 with GCC and C99. I am using -O3 here. stdlib seems to have all sorts of fun compiler optimizations at hand, and this example makes use of that (memcpy is actually compiled out here).
Do this by:
- (.rodata) FOO_ASSIGN_VAL is stored in .rodata
- (.text) a sequence of *movl FOO_ASSIGN_VAL, %registers* occur
- (.text) a sequence of movl %registers, foo occur
Say Foo was a 48 field struct of uint8_t values. It is aligned in memory.
( IDEAL ) On a 32-bit machine, this COULD be as quick as 12 MOVL instructions of immediates out to foo's address space. For me this is 12*10 == 120bytes of .text in size.
( ACTUAL ) However, using the answer by AUTO will likely generate 48 MOVB instructions in .text. For me this is 48*7 == 336bytes of .text!!
( SMALLEST* ) Use the memcpy version above. IF alignment is taken care of,
- FOO_ASSIGN_VAL is placed in .rodata (48 bytes),
- 12 MOVL into %register
- 12 MOVL outof %registers are used in .text (24*10) == 240bytes.
- For me then this is a total of 288 bytes.
So, for me at least with my i386 code,
*Smallest here means 'smallest footprint I know of'. It also executes faster than the above methods (24 instructions vs 48). Of course, the IDEAL version is fastest & smallest, but I still can't figure that out.
*Does anyone know how to get implementation of ' IDEAL ' above? It is annoying the hell out of me!!

If you don't care too much about efficiency, you could double assign: i.e. create a new instance of the structure using aggregate initialization, and then copy it over:
Make sure you keep the portion wrapped in {}s so as to discard the unnecessary temporary variable as soon as it's no longer necessary.
Note this isn't as efficient as making, e.g., a 'set' function in the struct (if c++) or out of the struct, accepting a struct pointer (if C). But if you need a quick, preferably temporary, alternative to writing element-by-element assignment, this might do.

- Also compilers will optimize that. – Pavel Ognev Commented May 8, 2013 at 8:05
- Why stop there? struct Foo foo_new(int bunch, int of, int things, int initialized) { struct Foo tmp = {bunch, of, things, initialized}; return tmp; }; struct Foo foo; foo = foo_new(1, 2, 3, 4); Stick it in a header file as inline and the performance impact should be minimal with optimization. The only downside is you can't leave out any things to be zeroed out at the end (though some may not consider that a downside given the existence of the missing-field-initializers warning). Of course, the (Foo){...} style is probably the simplest when it's available. – JAB Commented Mar 24, 2016 at 23:29
If you care about efficiency, you can define a union of the same length as your structure, with a type you can assign at once.
To assign values by elements use the struct of your union, to assign the whole data, use the other type of your union.
Be carefull about your memory organization (is "a" the MSB or the LSB of "whole"?).
Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged c++ c struct or ask your own question .
- The Overflow Blog
- The world’s largest open-source business has plans for enhancing LLMs
- Featured on Meta
- User activation: Learnings and opportunities
- Site maintenance - Mon, Sept 16 2024, 21:00 UTC to Tue, Sept 17 2024, 2:00...
- What does a new user need in a homepage experience on Stack Overflow?
- Announcing the new Staging Ground Reviewer Stats Widget
Hot Network Questions
- Understanding symmetry in a double integral
- The walk radical in Traditional Chinese
- Whom did Jesus' followers accompany -- a soldier or a civilian?
- Is it possible to draw this picture without lifting the pen? (I actually want to hang string lights this way in a gazebo without doubling up)
- how does the US justice system combat rights violations that happen when bad practices are given a new name to avoid old rulings?
- Swapping front Shimano 105 R7000 34x50t 11sp Chainset with Shimano Deore FC-M5100 chainset; 11-speed 26x36t
- Little spikes on mains AC
- Logic Test: Room 1
- Delete only faces in whole scene
- How to make a soundless world
- Would a scientific theory of everything be falsifiable?
- Is Entropy time-symmetric?
- тем и есть (syntax and meaning)
- Doesn't nonlocality follow from nonrealism in the EPR thought experiment and Bell tests?
- How to use Modus Ponens in L axiomatic system
- Is Sagittarius A* smaller than we might expect given the mass of the Milky Way?
- The consequence of a good letter of recommendation when things do not work out
- What is the oldest open math problem outside of number theory?
- What is the action-cost of grabbing spell components?
- Should I be careful about setting levels too high at one point in a track?
- Do I have to use a new background that's been republished under the 2024 rules?
- Latin lyrics to "Far away"
- If one is arrested, but has a baby/pet in their house, what are they supposed to do?
- Why is steaming food faster than boiling it?

IMAGES
VIDEO
COMMENTS
You can if the values are already part of a similar struct, that is, you can do this: Student s1 = {.id = id, .name = name, .score = score}; which creates an instance of Student and initializes the fields you specify. This probably isn't really any more efficient than assigning values individually, but it does keep the code concise.
4. Yes, you can assign one instance of a struct to another using a simple assignment statement. In the case of non-pointer or non pointer containing struct members, assignment means copy. In the case of pointer struct members, assignment means pointer will point to the same address of the other pointer.
To access the structure, you must create a variable of it. Use the struct keyword inside the main() method, followed by the name of the structure and then the name of the structure variable: Create a struct variable with the name "s1": struct myStructure {. int myNum; char myLetter; }; int main () {. struct myStructure s1;
In this tutorial, you'll learn about struct types in C Programming. You will learn to define and use structures with the help of examples. In C programming, a struct (or structure) is a collection of variables (can be of different types) under a single name.
15.13 Structure Assignment. Assignment operating on a structure type copies the structure. The left and right operands must have the same type. Here is an example: Notionally, assignment on a structure type works by copying each of the fields. Thus, if any of the fields has the const qualifier, that structure type does not allow assignment:
The structure in C is a user-defined data type that can be used to group items of possibly different types into a single type. The struct keyword is used to define the structure in the C programming language. The items in the structure are called its member and they can be of any valid data type. Additionally, the values of a structure are stored in contiguous memory locations.
structattr-spec-seq (optional)name. (2) 1) Struct definition: introduces the new type struct name and defines its meaning. 2) If used on a line of its own, as in structname;, declares but doesn't define the struct name (see forward declaration below). In other contexts, names the previously-declared struct, and attr-spec-seq is not allowed.
Instead of using the struct home_address every time you need to declare struct variable, you can simply use addr, the typedef that we have defined. You can read the typedef in detail here. Array of Structures in C. An array of structures is an array with structure as elements. For example: Here, stu[5] is an array of structures.
Code language: C++ (cpp) The address structure includes house number, street name, city, state, zip code, and country.. Declaring structure variables. The above example defines the address structure without declaring any structure variables.C provides you with two ways to declare structure variables: 1) Declaring structure variables with the structure definition
How to declare a struct variable; How to access and assign data to a struct property member; How to access and assign data to a struct pointer; Summary; What is a struct A struct is a user-defined type that can store one or more variables of different types. We use structs to model our application after entities in the real world, grouping ...
C Structure and Function; C Unions; C Programming Files. C File Handling; C Files Examples; C Additional Topics. C Keywords and Identifiers; ... In the above example, n number of struct variables are created where n is entered by the user. To allocate the memory for n number of struct person, we used, ptr = (struct person*) malloc(n * sizeof ...
As you can see in this example you are required to assign a value to all variables contained in your new data type. To access a structure variable you can use the point like in stu.name. There is also a shorter way to assign values to a structure: typedef struct{ int x; int y; }point; point image_dimension = {640,480};
Pointers to Structs. Part 1 contains C basics, including functions, static arrays, I/O. Links to other C programming Resources. C Stucts and Pointers. This is the second part of a two part introduction to the C programming language. It is written specifically for CS31 students. The first part covers C programs, compiling and running, variables ...
In C, a structure (struct) is a user-defined data type that allows us to combine data items of different data types into a single unit. In this article, we will learn how to initialize structures in C. Initialize Structures in C. We can initialize the structure by providing the values of the members in the list during the declaration.
Structures in C. A structure in C is a derived or user-defined data type. We use the keyword struct to define a custom data type that groups together the elements of different types. The difference between an array and a structure is that an array is a homogenous collection of similar types, whereas a structure can have elements of different types stored adjacently and identified by a name.
Passing struct by reference. You can also pass structs by reference (in a similar way like you pass variables of built-in type by reference). We suggest you to read pass by reference tutorial before you proceed.. During pass by reference, the memory addresses of struct variables are passed to the function.
struct (C programming language) In the C programming language, struct is the keyword used to define a composite, a.k.a. record, data type - a named set of values that occupy a block of memory. It allows for the different values to be accessed via a single identifier, often a pointer. A struct can contain other data types so is used for mixed ...
In C, the only operation that can be applied to struct variables is assignment. Any other operation (e.g. equality check) is not allowed on struct variables. ... Write a C program to show memory representation of C variables like int, float, pointer, etc. Algorithm: Get the address and size of the variable. ...
Assignment Operator; Destructor; Move Constructor (C++11) Move Assignment (C++11) If you want to know why? It is to maintain backward compatibility with C (because C structs are copyable using = and in declaration). But it also makes writing simple classes easier. Some would argue that it adds problems because of the "shallow copy problem".
The struct1=struct2; notation is not only more concise, but also shorter and leaves more optimization opportunities to the compiler. The semantic meaning of = is an assignment, while memcpy just copies memory. That's a huge difference in readability as well, although memcpy does the same in this case. Use =.
In C++11 you can perform multiple assignment with "tie" (declared in the tuple header) struct foo {. int a, b, c; } f; std::tie(f.a, f.b, f.c) = std::make_tuple(1, 2, 3); If your right hand expression is of fixed size and you only need to get some of the elements, you can use the ignore placeholder with tie.